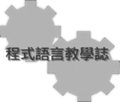
encrypt.py 的程式原始碼如下
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 | import random class Encrypt: def __init__( self ): self .code = [ chr (i) for i in range ( 97 , 123 )] random.shuffle( self .code) self .alph = [ chr (i) for i in range ( 97 , 123 )] def __str__( self ): return "code: " + "".join( self .code) def setCode( self , data): self .code = list (data) def getCode( self ): return "".join( self .code) def toEncode( self , s): result = "" for i in s: if i in self .code: j = self .alph.index(i) result + = self .code[j] else : result + = i return result def toDecode( self , s): result = "" for i in s: if i in self .code: j = self .code.index(i) result + = self .alph[j] else : result + = i return result if __name__ = = '__main__' : e = Encrypt() print () print (e) s1 = "There is no spoon." print ( "input: " + s1) s2 = e.toEncode(s1) print ( "encode: " + s2) s3 = e.toDecode(s2) print ( "decode: " + s3) print () # 《程式語言教學誌》的範例程式 # 檔名:encrypt.py # 功能:示範 Python 程式 # 作者:張凱慶 # 時間:西元 2012 年 12 月 |
您可以繼續參考
範例程式碼
相關目錄
回 Python 入門指南
回 Python 教材
回首頁
參考資料
http://www.python.org/
http://docs.python.org/3.2/tutorial/index.html
http://docs.python.org/3.2/library/index.html
http://www.tutorialspoint.com/python/index.htm
http://interactivepython.org/courselib/static/thinkcspy/index.html
http://interactivepython.org/courselib/static/pythonds/index.html
http://en.wikibooks.org/wiki/Python_Programming
http://jwork.org/learn/doc/doku.php?id=python:start
沒有留言:
張貼留言