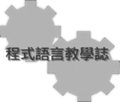
encryptgui.rb 的程式原始碼如下
require 'tk' require './encrypt.rb' class EncryptGUI def initialize # 每個 command 的設定 p_new = proc {newMethod} p_load = proc {loadMethod} p_save = proc {saveMethod} p_encode = proc {encodeMethod} p_decode = proc {decodeMethod} p_clear = proc {clearMethod} p_copy = proc {copyMethod} p_input = proc {inputMethod} # 設定實體變數的初值 @e = nil @userinput = "" @result = "" # 設定 GUI 各元件 root = TkRoot.new { title "EncryptGUI Demo" } @inputText = TkLabel.new(root) { text "Input:" width 8 height 1 grid('row'=>0, 'column'=>0) } @inputField = TkEntry.new(root) { width 60 grid('row'=>0, 'column'=>1, 'columnspan'=>6) } @outputText = TkLabel.new(root) { text 'Output:' width 8 height 1 grid('row'=>1, 'column'=>0) } @outputField = TkEntry.new(root) { width 60 grid('row'=>1, 'column'=>1, 'columnspan'=>6) } @newButton = TkButton.new(root) { text "New" grid('row'=>2, 'column'=>0) command p_new } @loadButton = TkButton.new(root) { text "Load" grid('row'=>2, 'column'=>1) command p_load } @saveButton = TkButton.new(root) { text "Save" grid('row'=>2, 'column'=>2) command p_save } @encodeButton = TkButton.new(root) { text "Encode" grid('row'=>2, 'column'=>3) command p_encode } @decodeButton = TkButton.new(root) { text "Decode" grid('row'=>2, 'column'=>4) command p_decode } @clearButton = TkButton.new(root) { text "Clear" grid('row'=>2, 'column'=>5) command p_clear } @copyButton = TkButton.new(root) { text "Copy" grid('row'=>2, 'column'=>6) command p_copy } @displayText = TkLabel.new(root) { text 'something happened' width 65 height 1; justify 'left' grid('row'=>4, 'column'=>0, 'columnspan'=>7) } end # 建立新 Encrypt 物件 def newMethod @e = Encrypt.new @displayText.text = "code: #{@e.getCode}" end # 載入儲存的密碼表 def loadMethod if File.exist?("./code.txt") _arr = IO.readlines("./code.txt") @e = Encrypt.new @e.setCode(_arr[0]) @displayText.text = "load: #{@e.getCode}" else @displayText.text = "Load denied!!" end end # 儲存密碼表 def saveMethod if @e == nil @displayText.text = "No Encrypt object can save!!" else _file = File.new("./code.txt", "w") _file.syswrite(@e.getCode) @displayText.text = "The code is saved." end end # 進行編碼 def encodeMethod @userinput = @inputField.value if @userinput == "" @displayText.text = "No input string!!" else if @e == nil @displayText.text = "No encrypt object!!" else @result = @e.toEncode(@userinput) @outputField.value = @result @displayText.text = "Encoding success!!" end end end # 進行解碼 def decodeMethod @userinput = @inputField.value if @userinput == "" @displayText.text = "No input string!!" else if @e == nil @displayText.text = "No encrypt object!!" else @result = @e.toDecode(@userinput) @outputField.value = @result @displayText.text = "Decoding success!!" end end end # 清除所有輸入、輸出 def clearMethod @e = nil @userinput = "" @result = "" @inputField.value = "" @outputField.value = "" @displayText.text = "It's done." end # 拷貝編碼結果到剪貼簿 def copyMethod if @result == "" @displayText.text = "Copy denied!!" else TkClipboard.set(@result) @displayText.text = "It is already copied to the clipboard." end end end =begin 《程式語言教學誌》的範例程式 http://pydoing.blogspot.com/ 檔名:encryptgui.rb 功能:示範 Ruby 程式 作者:張凱慶 時間:西元 2012 年 12 月 =end
您可以繼續參考
範例程式碼
相關目錄
回 Ruby 入門指南
回 Ruby 教材
回首頁
參考資料
http://www.ruby-lang.org/en/
http://www.ruby-lang.org/en/documentation/
http://rubylearning.com/
http://www.techotopia.com/index.php/Ruby_Essentials
http://pine.fm/LearnToProgram/
http://ruby-doc.org/docs/ProgrammingRuby/
http://www.tutorialspoint.com/ruby/index.htm
http://www.rubyist.net/~slagell/ruby/
http://en.wikibooks.org/wiki/Ruby_programming_language
http://www.ruby-doc.org/core-1.9.3/
http://www.ruby-doc.org/stdlib-1.9.3/
沒有留言:
張貼留言