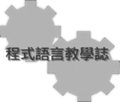
encryptgui.cs 的程式原始碼如下
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186 187 188 189 190 191 192 193 194 195 196 197 198 199 200 201 202 203 204 205 206 207 208 209 210 211 212 213 214 215 216 217 218 219 220 221 222 223 224 225 226 227 228 229 230 231 232 233 234 235 236 237 238 239 240 241 242 | using System; using System.Windows.Forms; using System.Drawing; using System.IO; using System.Text; using Encryptor; class EncryptGUI : Form { private Label inputLabel; private Label outputLabel; private TextBox inputField; private TextBox outputField; private Button newButton; private Button loadButton; private Button saveButton; private Button encodeButton; private Button decodeButton; private Button clearButton; private Button copyButton; private Label displayLabel; private string inputText; private string outputText; private Encrypt e; public EncryptGUI() { this .inputLabel = new Label(); this .outputLabel = new Label(); this .inputField = new TextBox(); this .outputField = new TextBox(); this .newButton = new Button(); this .loadButton = new Button(); this .saveButton = new Button(); this .encodeButton = new Button(); this .decodeButton = new Button(); this .clearButton = new Button(); this .copyButton = new Button(); this .displayLabel = new Label(); this .SuspendLayout(); this .inputLabel.Location = new Point(10, 10); this .inputLabel.Font = new Font( "Helvetica" , 12F); this .inputLabel.Name = "inputlabel" ; this .inputLabel.Size = new Size(60, 20); this .inputLabel.Text = "Input:" ; this .outputLabel.Location = new Point(10, 40); this .outputLabel.Font = new Font( "Helvetica" , 12F); this .outputLabel.Name = "outputlabel" ; this .outputLabel.Size = new Size(60, 20); this .outputLabel.Text = "Output:" ; this .inputField.Location = new Point(80, 10); this .inputField.Name = "inputField" ; this .inputField.Size = new Size(480, 20); this .inputField.TabIndex = 0; this .inputField.Text = "" ; this .inputField.KeyPress += new KeyPressEventHandler( this .inputField_KeyPress); this .outputField.Location = new Point(80, 40); this .outputField.Name = "outputField" ; this .outputField.Size = new Size(480, 20); this .outputField.Enabled = false ; this .outputField.Text = "" ; this .newButton.Location = new Point(10, 70); this .newButton.Name = "newButton" ; this .newButton.TabIndex = 1; this .newButton.Text = "New" ; this .newButton.Click += new EventHandler( this .newButton_Click); this .loadButton.Location = new Point(90, 70); this .loadButton.Name = "loadButton" ; this .loadButton.TabIndex = 2; this .loadButton.Text = "Load" ; this .loadButton.Click += new EventHandler( this .loadButton_Click); this .saveButton.Location = new Point(170, 70); this .saveButton.Name = "saveButton" ; this .saveButton.TabIndex = 3; this .saveButton.Text = "Save" ; this .saveButton.Click += new EventHandler( this .saveButton_Click); this .encodeButton.Location = new Point(250, 70); this .encodeButton.Name = "encodeButton" ; this .encodeButton.TabIndex = 4; this .encodeButton.Text = "Encode" ; this .encodeButton.Click += new EventHandler( this .encodeButton_Click); this .decodeButton.Location = new Point(330, 70); this .decodeButton.Name = "decodeButton" ; this .decodeButton.TabIndex = 5; this .decodeButton.Text = "Decode" ; this .decodeButton.Click += new EventHandler( this .decodeButton_Click); this .clearButton.Location = new Point(410, 70); this .clearButton.Name = "clearButton" ; this .clearButton.TabIndex = 6; this .clearButton.Text = "Clear" ; this .clearButton.Click += new EventHandler( this .clearButton_Click); this .copyButton.Location = new Point(490, 70); this .copyButton.Name = "copyButton" ; this .copyButton.TabIndex = 7; this .copyButton.Text = "Copy" ; this .copyButton.Click += new EventHandler( this .copyButton_Click); this .displayLabel.Location = new Point(10, 100); this .displayLabel.Font = new Font( "Courier" , 12F); this .displayLabel.Name = "displaylabel" ; this .displayLabel.Size = new Size(500, 20); this .displayLabel.Text = "something happened..." ; this .ClientSize = new Size(580, 125); this .Controls.Add( this .inputLabel); this .Controls.Add( this .outputLabel); this .Controls.Add( this .inputField); this .Controls.Add( this .outputField); this .Controls.Add( this .newButton); this .Controls.Add( this .loadButton); this .Controls.Add( this .saveButton); this .Controls.Add( this .encodeButton); this .Controls.Add( this .decodeButton); this .Controls.Add( this .clearButton); this .Controls.Add( this .copyButton); this .Controls.Add( this .displayLabel); this .BackColor = Color.LightGray; this .Name = "demoguiname" ; this .Text = "demogui" ; this .inputText = "" ; this .outputText = "" ; this .e = null ; this .ResumeLayout( false ); } private void inputField_KeyPress( object sender, EventArgs e) { this .inputText = this .inputField.Text; this .displayLabel.Text = "Your input is \"" + this .inputText + "\"." ; } private void newButton_Click( object sender, EventArgs e) { this .e = new Encrypt(); this .displayLabel.Text = this .e.Code; } private void loadButton_Click( object sender, EventArgs e) { string path = @"data.txt" ; if (File.Exists(path)) { try { string code = File.ReadAllText(path, Encoding.UTF8); this .e.Code = code; this .displayLabel.Text = "This is Load button. Encrypt object is loaded." ; } catch (NullReferenceException nre) { this .displayLabel.Text = "This is Load button. Encrypt object is not loaded." ; } } else { this .displayLabel.Text = "This is Load button. There is no file." ; } } private void saveButton_Click( object sender, EventArgs e) { string path = @"data.txt" ; try { File.WriteAllText(path, this .e.Code); this .displayLabel.Text = "This is Save button. Encrypt object is saved." ; } catch (NullReferenceException nre) { this .displayLabel.Text = "This is Save button. There is no Encrypt Object." ; } } private void encodeButton_Click( object sender, EventArgs e) { if ( this .inputText == "" ) { this .displayLabel.Text = "This is Encode button. No input string!!" ; } else { try { this .outputText = this .e.toEncode( this .inputText); this .outputField.Text = this .outputText; this .displayLabel.Text = "This is Encode button. The result is above." ; } catch (NullReferenceException nre) { this .displayLabel.Text = "This is Encode button. No Encrypt object!!" ; } } } private void decodeButton_Click( object sender, EventArgs e) { if ( this .inputText == "" ) { this .displayLabel.Text = "This is Decode button. No input string!!" ; } else { try { this .outputText = this .e.toDecode( this .inputText); this .outputField.Text = this .outputText; this .displayLabel.Text = "This is Decode button. The result is above." ; } catch (NullReferenceException nre) { this .displayLabel.Text = "This is Decode button. No Encrypt object!!" ; } } } private void clearButton_Click( object sender, EventArgs e) { this .inputText = "" ; this .outputText = "" ; this .inputField.Text = "" ; this .outputField.Text = "" ; this .displayLabel.Text = "This is Clear button. Everything is clear." ; } private void copyButton_Click( object sender, EventArgs e) { if ( this .outputText != "" ) { Clipboard.SetText( this .outputText); this .displayLabel.Text = "This is Copy button. Result is copied." ; } else { this .displayLabel.Text = "This is Copy button. Result is not copied." ; } } [STAThread] public static void Main() { Application.EnableVisualStyles(); Application.Run( new EncryptGUI()); } } /* 《程式語言教學誌》的範例程式 檔名:encryptgui.cs 功能:示範 C# 程式 作者:張凱慶 時間:西元 2012 年 10 月 */ |
您可以繼續參考
範例程式碼
相關目錄
回 C# 入門指南
回 C# 教材
回首頁
參考資料
C# 程式設計手冊
C# Programming
Start - mono
Mono Documentation
沒有留言:
張貼留言