a = 22 #1 print(a.__str__()) print(type(a.__str__())) print() a = 22.0 #2 print(a.__str__()) print(type(a.__str__())) print() a = "22" #3 print(a.__str__()) print(type(a.__str__())) print() a = [22] #4 print(a.__str__()) print(type(a.__str__())) # 《程式語言教學誌》的範例程式 # http://pydoing.blogspot.com/ # 檔名:cla06.py # 功能:示範 Python 程式 # 作者:張凱慶 # 時間:西元 2010 年 12 月
執行結果如下
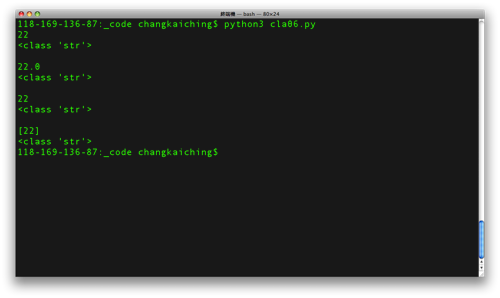
第 1 組
a = 22 #1 print(a.__str__()) print(type(a.__str__()))
a 為整數 (integer) ,其字串形式如同 "22" 。
第 2 組
a = 22.0 #2 print(a.__str__()) print(type(a.__str__()))
a 為浮點數 (floating-point number) ,其字串形式如同 "22.0" 。
第 3 組
a = "22" #3 print(a.__str__()) print(type(a.__str__()))
a 就是字串,字串形式也就是 "22" 。
第 4 組
a = [22] #4 print(a.__str__()) print(type(a.__str__()))
a 為具有一個整數 22 的串列 (list) ,其字串形式如同 "[22]" 。
自行定義的類別預設的字串表達形式如下例
class Demo: def __init__(self, i): self.i = i def hello(self): print("hello", self.i) a = Demo(1122) a.hello() print() print(a.__str__()) # 《程式語言教學誌》的範例程式 # http://pydoing.blogspot.com/ # 檔名:cla07.py # 功能:示範 Python 程式 # 作者:張凱慶 # 時間:西元 2010 年 12 月
執行結果如下
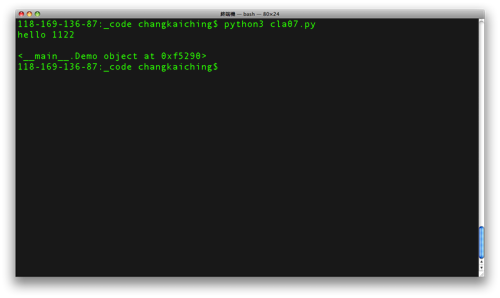
由於預設的方式是印出類別名稱,實體所在的記憶體位址,這未必是我們想要的,因此我們可以視需要改寫 (override) __str__() 方法,如下
class Demo: def __init__(self, i): self.i = i def __str__(self): return str(self.i) def hello(self): print("hello", self.i) a = Demo(1122) a.hello() print() print(a.__str__()) # 《程式語言教學誌》的範例程式 # http://pydoing.blogspot.com/ # 檔名:cla08.py # 功能:示範 Python 程式 # 作者:張凱慶 # 時間:西元 2010 年 12 月
執行結果如下
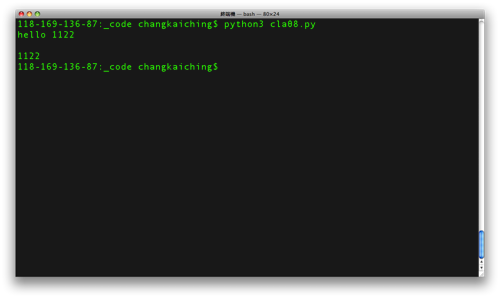
第 5 行到第 6 行
def __str__(self): return str(self.i)
我們簡單的讓 Demo 的 __str__() 回傳一個運算式 (expression) ,這個運算式為以實體屬性 (attribute) self.i 當成參數 (parameter) ,呼叫 (call) 內建函數 (function) str() , str() 回傳包含該數值的字串物件。
這裡,由於我們將 a 的 self.i 設定成整數 1122 ,因此字串形式為 "1122" 。
中英文術語對照 | |
---|---|
類別 | class |
方法 | method |
實體 | instance |
物件 | object |
字串 | string |
資料型態 | data type |
整數 | integer |
浮點數 | floating-point number |
串列 | list |
改寫 | override |
運算式 | expression |
屬性 | attribute |
參數 | parameter |
呼叫 | call |
函數 | function |
參考資料
http://docs.python.org/py3k/tutorial/classes.html
http://docs.python.org/py3k/reference/compound_stmts.html
http://docs.python.org/py3k/tutorial/classes.html
http://docs.python.org/py3k/reference/compound_stmts.html
沒有留言:
張貼留言