運算子 | 功能 | 範例 |
---|---|---|
+ | 正 | +a |
- | 負 | -a |
++ | 遞增 | ++a, a++ |
-- | 遞減 | --a, a-- |
! | 邏輯補數運算子 | ~a |
其中,邏輯補數運算子運用在布林型態,也就是利用關鍵字 (keyword) boolean 所宣告的變數 (variable) ,其餘皆可運算在基本資料型態 (primitive data type) 中的算術型態。以下為整數型態 (integral type) 的例子
class UnaryIntDemo { public static void main(String[] args) { int a, c; a = 8; c = +a; System.out.println("+a = " + c); c = -a; System.out.println("-a = " + c); c = ++a; System.out.println("++a = " + c); c = --a; System.out.println("--a = " + c); } } /* 《程式語言教學誌》的範例程式 http://pydoing.blogspot.com/ 檔名:UnaryIntDemo.java 功能:示範單元運算子的使用 作者:張凱慶 時間:西元 2010 年 10 月 */
編譯後執行,結果如下
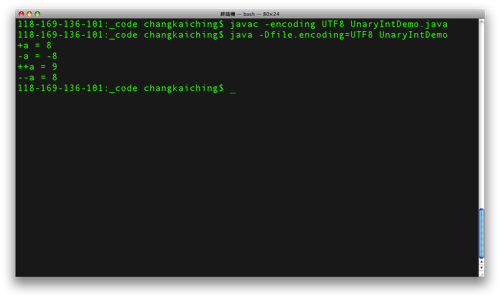
以下為浮點數型態 (floating-point type) 做單元運算的例子
class UnaryFloatDemo { public static void main(String[] args) { double a, c; a = 8.3; c = +a; System.out.println("+a = " + c); c = -a; System.out.println("-a = " + c); c = ++a; System.out.println("++a = " + c); c = --a; System.out.println("--a = " + c); } } /* 《程式語言教學誌》的範例程式 http://pydoing.blogspot.com/ 檔名:UnaryFloatDemo.java 功能:示範單元運算子的使用 作者:張凱慶 時間:西元 2010 年 10 月 */
編譯後執行,結果如下
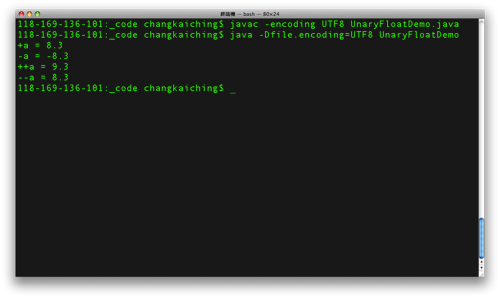
以下為布林型態使用邏輯補數運算子的例子
class UnaryBooleanDemo { public static void main(String[] args) { boolean b, c; b = true; c = !b; System.out.println("!b = " + c); } } /* 《程式語言教學誌》的範例程式 http://pydoing.blogspot.com/ 檔名:UnaryBooleanDemo.java 功能:示範單元運算子的使用 作者:張凱慶 時間:西元 2010 年 10 月 */
編譯後執行,結果如下
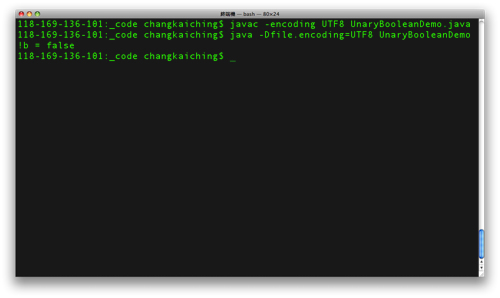
字元型態也可以遞增遞減運算子,例如以下程式依序印出 26 個英文小寫字母
class UnaryCharDemo { public static void main(String[] args) { char b, c; b = 'a'; c = b; while (c <= 'z') { System.out.print(c); c++; } System.out.println(); } } /* 《程式語言教學誌》的範例程式 http://pydoing.blogspot.com/ 檔名:UnaryCharDemo.java 功能:示範單元運算子的使用 作者:張凱慶 時間:西元 2010 年 10 月 */
編譯後執行,結果如下
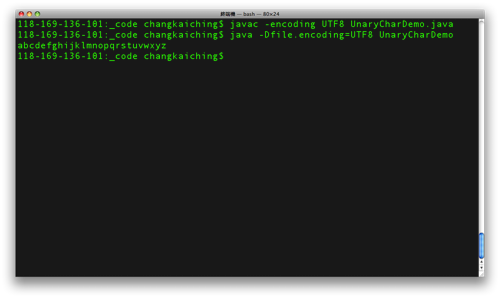
中英文術語對照 | |
---|---|
單元運算子 | unary operator |
運算元 | operand |
遞增運算子 | increment operator |
遞減運算子 | decrement operator |
邏輯補數運算子 | logical compliment operator |
關鍵字 | keyword |
變數 | variable |
基本資料型態 | primitive data type |
整數型態 | integral type |
浮點數型態 | floating-point type |
參考資料
http://download.oracle.com/javase/tutorial/java/nutsandbolts/op1.html
http://java.sun.com/docs/books/jls/third_edition/html/expressions.html
http://download.oracle.com/javase/tutorial/java/nutsandbolts/op1.html
http://java.sun.com/docs/books/jls/third_edition/html/expressions.html
沒有留言:
張貼留言