運算子 | 功能 | 範例 |
---|---|---|
== | 相等 | a == b |
!= | 不相等 | a != b |
> | 大於 | a >= b |
>= | 大於等於 | a >= b |
< | 小於 | a < b |
<= | 小於等於 | a <= b |
Java 使用連續兩個等號 == 測試相等性,注意這與一般我們手寫的數學等號 = ,這不一樣喔!
基本資料型態 (primitive data type) 皆可運用相等性及關係運子,以下為整數型態 (integral type) 的例子
class RelationIntDemo { public static void main(String[] args) { int a = 12; int b = 22; if (a < b) { System.out.println("a < b\n"); } if (a <= b) { System.out.println("a <= b\n"); } if (a > b) { System.out.println("a > b\n"); } if (a >= b) { System.out.println("a >= b\n"); } if (a == b) { System.out.println("a == b\n"); } if (a != b) { System.out.println("a != b\n"); } } } /* 《程式語言教學誌》的範例程式 http://pydoing.blogspot.com/ 檔名:RelationIntDemo.java 功能:示範相等性及關係運算子的使用 作者:張凱慶 時間:西元 2010 年 10 月 */
編譯後執行,結果如下
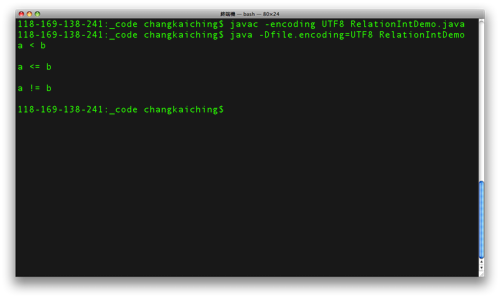
以下為浮點數型態 (floating-point type) 做單元運算的例子
class RelationFloatDemo { public static void main(String[] args) { double a = 22.0; double b = 22.0; if (a < b) { System.out.println("a < b"); } if (a <= b) { System.out.println("a <= b"); } if (a > b) { System.out.println("a > b"); } if (a >= b) { System.out.println("a >= b"); } if (a == b) { System.out.println("a == b"); } if (a != b) { System.out.println("a != b"); } } } /* 《程式語言教學誌》的範例程式 http://pydoing.blogspot.com/ 檔名:RelationFloatDemo.java 功能:示範相等性及關係運算子的使用 作者:張凱慶 時間:西元 2010 年 10 月 */
編譯後執行,結果如下
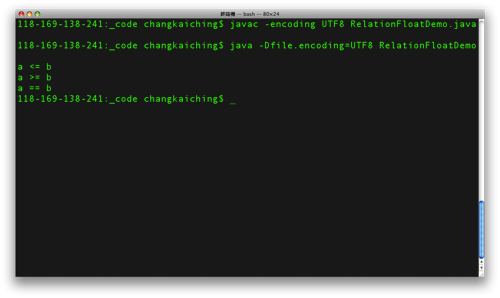
以下為布林型態的例子
class RelationBooleanDemo { public static void main(String[] args) { boolean a = true; boolean b = false; if (a == b) { System.out.println("a == b"); } if (a != b) { System.out.println("a != b"); } } } /* 《程式語言教學誌》的範例程式 http://pydoing.blogspot.com/ 檔名:RelationBoolDemo.java 功能:示範單元運算子的使用 作者:張凱慶 時間:西元 2010 年 10 月 */
編譯後執行,結果如下
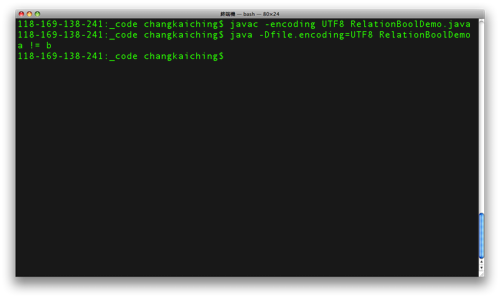
以下為字元型態的例子
class RelationCharDemo { public static void main(String[] args) { char a = 'A'; char b = 'a'; if (a < b) { System.out.println("a < b"); } if (a <= b) { System.out.println("a <= b"); } if (a > b) { System.out.println("a > b"); } if (a >= b) { System.out.println("a >= b"); } if (a == b) { System.out.println("a == b"); } if (a != b) { System.out.println("a != b"); } } } /* 《程式語言教學誌》的範例程式 http://pydoing.blogspot.com/ 檔名:RelationCharDemo.java 功能:示範單元運算子的使用 作者:張凱慶 時間:西元 2010 年 10 月 */
編譯後執行,結果如下
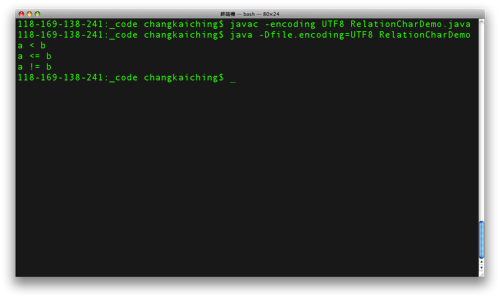
中英文術語對照 | |
---|---|
關係運算子 | equality and relational operator |
布林字面常數 | Boolean literal |
基本資料型態 | primitive data type |
整數型態 | integral type |
浮點數型態 | floating-point type |
參考資料
http://download.oracle.com/javase/tutorial/java/nutsandbolts/op2.html
http://java.sun.com/docs/books/jls/third_edition/html/expressions.html
http://download.oracle.com/javase/tutorial/java/nutsandbolts/op2.html
http://java.sun.com/docs/books/jls/third_edition/html/expressions.html
沒有留言:
張貼留言