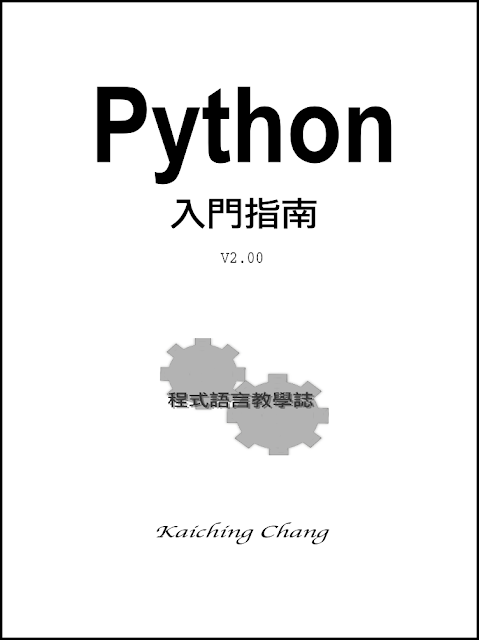
001 | from random import shuffle |
002 | |
003 | # 定義 Encrypt 類別 |
004 | class Encrypt: |
005 | # 建立 Encrypt 物件同時建立密碼表 |
006 | def __init__(self, str=None): |
007 | # 設定 code |
008 | if str == None: |
009 | self.code = [chr(i) for i in |
010 | range(97, 123)] |
011 | shuffle(self.code) |
012 | else: |
013 | self.code = list(str) |
014 | |
015 | # 設定 alph |
016 | self.alph = [chr(i) for i in |
017 | range(97, 123)] |
018 | |
019 | # 回傳密碼表字串 |
020 | def __str__(self): |
021 | code = "".join(self.code) |
022 | return "code: " + code |
023 | |
024 | # 編碼的方法 |
025 | def toEncode(self, str): |
026 | # 暫存編碼結果的字串 |
027 | result = "" |
028 | |
029 | # 利用迴圈走完參數字串的所有字元 |
030 | for i in str: |
031 | # 判斷該字元是否為英文小寫字母 |
032 | # 若是英文小寫字母就進行編碼轉換 |
033 | if i in self.code: |
034 | j = self.alph.index(i) |
035 | result += self.code[j] |
036 | else: |
037 | result += i |
038 | |
039 | # 結束回傳編碼過的字串 |
040 | return result |
041 | |
042 | # 解碼的方法 |
043 | def toDecode(self, str): |
044 | # 暫存解碼結果的字串 |
045 | result = "" |
046 | |
047 | # 利用迴圈走完參數字串的所有字元 |
048 | for i in str: |
049 | # 判斷該字元是否為英文小寫字母 |
050 | # 若是英文小寫字母就進行解碼轉換 |
051 | if i in self.code: |
052 | j = self.code.index(i) |
053 | result += self.alph[j] |
054 | else: |
055 | result += i |
056 | |
057 | # 結束回傳解碼過的字串 |
058 | return result |
059 | |
060 | # 測試部分 |
061 | if __name__ == '__main__': |
062 | e = Encrypt() |
063 | print() |
064 | print(e) |
065 | s1 = "There is no spoon." |
066 | print("Input : " + s1) |
067 | s2 = e.toEncode(s1) |
068 | print("Encode: " + s2) |
069 | s3 = e.toDecode(s2) |
070 | print("Decode: " + s3) |
071 | print() |
072 | |
073 | # 檔名: encrypt.py |
074 | # 作者: Kaiching Chang |
075 | # 時間: July, 2014 |
the end
沒有留言:
張貼留言