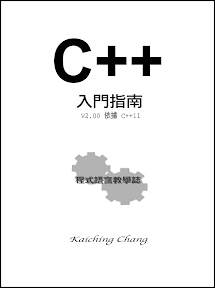
001 | #include "encryptwindow.h" |
002 | #include "ui_encryptwindow.h" |
003 | |
004 | #include <QFile> |
005 | #include <QDataStream> |
006 | #include <QClipboard> |
007 | |
008 | // 建構函數 |
009 | EncryptWindow::EncryptWindow(QWidget *parent) : |
010 | QMainWindow(parent), |
011 | ui(new Ui::EncryptWindow), |
012 | e(NULL) |
013 | { |
014 | ui->setupUi(this); |
015 | } |
016 | |
017 | // 解構函數 |
018 | EncryptWindow::~EncryptWindow() |
019 | { |
020 | delete ui; |
021 | delete e; |
022 | } |
023 | |
024 | // 將 string 轉換成 QString |
025 | QString EncryptWindow::s2q(const string &s) { |
026 | return QString(QString::fromLocal8Bit(s.c_str())); |
027 | } |
028 | |
029 | // 將 QString 轉換成 string |
030 | string EncryptWindow::q2s(const QString &s) { |
031 | return string((const char *)s.toLocal8Bit()); |
032 | } |
033 | |
034 | // 按下 New 按鈕的事件 |
035 | void EncryptWindow::on_pushButton_new_clicked() |
036 | { |
037 | e = new Encrypt(); |
038 | ui->label_display->setText(s2q(e->get_code_array())); |
039 | } |
040 | |
041 | // 按下 Save 按鈕的事件 |
042 | void EncryptWindow::on_pushButton_save_clicked() |
043 | { |
044 | // 先測試是否有按過 New 按鈕 |
045 | if (e != NULL) { |
046 | // 有按過 New 按鈕,建立檔名 encryptor 的 QFile 物件 |
047 | QFile file("encryptor"); |
048 | // 以寫入模式開啟檔案 |
049 | file.open(QIODevice::WriteOnly); |
050 | // 建立 QDataStream 物件讀取檔案串流 |
051 | QDataStream out(&file); |
052 | // 從 QDataStream 物件將密碼表輸出到檔案 |
053 | out << s2q(e->get_code_array()); |
054 | |
055 | // 最後在 label_display 顯示提示訊息 |
056 | ui->label_display->setText("Encrypt object is saved."); |
057 | } |
058 | else { |
059 | // 沒按過 New 按鈕,在 label_display 顯示提示訊息 |
060 | ui->label_display->setText("There is no Encrypt Object."); |
061 | } |
062 | } |
063 | |
064 | // 按下 Load 按鈕的事件 |
065 | void EncryptWindow::on_pushButton_load_clicked() |
066 | { |
067 | // 建立檔名 encryptor 的 QFile 物件 |
068 | QFile file("encryptor"); |
069 | // 以唯讀模式開啟,先測試檔案存不存在 |
070 | if (file.open(QIODevice::ReadOnly)) { |
071 | // 檔案存在,建立 QDataStream 物件讀取檔案串流 |
072 | QDataStream in(&file); |
073 | // 建立一個 QString 字串暫存密碼表 |
074 | QString temp; |
075 | // 從 QDataStream 物件將密碼表輸出到 QString 字串 |
076 | in >> temp; |
077 | |
078 | // 如果使用者沒按過 New ,先新建成員變數 e |
079 | if (e == NULL) { |
080 | e = new Encrypt; |
081 | } |
082 | // 將密碼表寫入成員變數 e |
083 | e->set_code_array(q2s(temp)); |
084 | |
085 | // 最後在 label_display 顯示提示訊息 |
086 | ui->label_display->setText("Encrypt object is loaded."); |
087 | } |
088 | else { |
089 | // 檔案不存在,在 label_display 顯示提示訊息 |
090 | ui->label_display->setText("Encrypt object is not loaded."); |
091 | } |
092 | } |
093 | |
094 | // 按下 Encode 按鈕的事件 |
095 | void EncryptWindow::on_pushButton_encode_clicked() |
096 | { |
097 | // 取得使用者輸入的英文句子 |
098 | input_text = ui->lineEdit_input->text(); |
099 | |
100 | // 先測試使用者是否有輸入 |
101 | if (input_text == ""){ |
102 | // 使用者沒有輸入,在 label_display 顯示提示訊息 |
103 | ui->label_display->setText("No input string!!"); |
104 | } |
105 | else { |
106 | // 使用者有輸入,測試使用者是否有按過 New 按鈕 |
107 | if (e == NULL) { |
108 | // 沒按過 New 按鈕,在 label_display 顯示提示訊息 |
109 | ui->label_display->setText("No Encrypt object!!"); |
110 | } |
111 | else { |
112 | // 有按過 New 按鈕,進行編碼工作並將結果顯示在 lineEdit_output |
113 | output_text = s2q(e->ToEncode(q2s(input_text))); |
114 | ui->lineEdit_output->setText(output_text); |
115 | ui->label_display->setText("The result is above."); |
116 | } |
117 | } |
118 | } |
119 | |
120 | // 按下 Decode 按鈕的事件 |
121 | void EncryptWindow::on_pushButton_decode_clicked() |
122 | { |
123 | // 取得使用者輸入的英文句子 |
124 | input_text = ui->lineEdit_input->text(); |
125 | |
126 | // 先測試使用者是否有輸入 |
127 | if (input_text == ""){ |
128 | // 使用者沒有輸入,在 label_display 顯示提示訊息 |
129 | ui->label_display->setText("No input string!!"); |
130 | } |
131 | else { |
132 | // 使用者有輸入,測試使用者是否有按過 New 按鈕 |
133 | if (e == NULL) { |
134 | // 沒按過 New 按鈕,在 label_display 顯示提示訊息 |
135 | ui->label_display->setText("No Encrypt object!!"); |
136 | } |
137 | else { |
138 | // 有按過 New 按鈕,進行編碼工作並將結果顯示在 lineEdit_output |
139 | output_text = s2q(e->ToDecode(q2s(input_text))); |
140 | ui->lineEdit_output->setText(output_text); |
141 | ui->label_display->setText("The result is above."); |
142 | } |
143 | } |
144 | } |
145 | |
146 | // 按下 Clear 按鈕的事件 |
147 | void EncryptWindow::on_pushButton_clear_clicked() |
148 | { |
149 | // 將暫存變數及輸出入欄位都設成空字串 |
150 | input_text = ""; |
151 | output_text = ""; |
152 | ui->lineEdit_input->setText(""); |
153 | ui->lineEdit_output->setText(""); |
154 | |
155 | ui->label_display->setText("Clear all!!"); |
156 | } |
157 | |
158 | // 按下 Copy 按鈕的事件 |
159 | void EncryptWindow::on_pushButton_copy_clicked() |
160 | { |
161 | // QClipboard 物件將文字拷貝到系統剪貼簿 |
162 | QClipboard *clipboard = QApplication::clipboard(); |
163 | clipboard->setText(output_text); |
164 | ui->label_display->setText("The result is copied to clipboard."); |
165 | |
166 | } |
167 | |
168 | // 輸入文字的事件 |
169 | void EncryptWindow::on_lineEdit_input_textChanged(const QString &arg1) |
170 | { |
171 | ui->label_display->setText("Your input is \"" + arg1 + "\"."); |
172 | } |
the end
沒有留言:
張貼留言