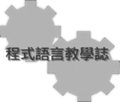
EncryptorGUI.java 的程式原始碼如下
import java.awt.*; import java.awt.event.*; import java.util.*; import javax.swing.*; import javax.swing.event.*; import encryptor.Encrypt; import java.io.*; public class EncryptorGUI { // 屬性區 private JFrame frame; private ArrayList<JComponent> GUIComponent; private String[] name; private int att[][]; private Encrypt e; private String userinput; private String result; // 設定屬性的建構子 public EncryptorGUI() { // fill 、 anchor 、 n 為常數 int fill[] = { GridBagConstraints.BOTH, GridBagConstraints.VERTICAL, GridBagConstraints.HORIZONTAL, GridBagConstraints.NONE}; int anchor[] = { GridBagConstraints.CENTER, GridBagConstraints.EAST, GridBagConstraints.SOUTHEAST, GridBagConstraints.SOUTH, GridBagConstraints.SOUTHWEST, GridBagConstraints.WEST, GridBagConstraints.NORTHWEST, GridBagConstraints.NORTH, GridBagConstraints.NORTHEAST}; int a[][] = {{0, 0, 1, 1, 0, 0, fill[3], anchor[5]}, {0, 1, 1, 1, 0, 0, fill[3], anchor[5]}, {0, 3, 7, 1, 0, 0, fill[3], anchor[5]}, {1, 0, 6, 1, 0, 0, fill[0], anchor[5]}, {1, 1, 6, 1, 0, 0, fill[0], anchor[5]}, {0, 2, 1, 1, 0, 0, fill[0], anchor[0]}, {1, 2, 1, 1, 0, 0, fill[0], anchor[0]}, {2, 2, 1, 1, 0, 0, fill[0], anchor[0]}, {3, 2, 1, 1, 0, 0, fill[0], anchor[0]}, {4, 2, 1, 1, 0, 0, fill[0], anchor[0]}, {5, 2, 1, 1, 0, 0, fill[0], anchor[0]}, {6, 2, 1, 1, 0, 0, fill[0], anchor[0]}}; String n[] = {"Input", "Output", "hint...", "New", "Load", "Save", "Encode", "Decode", "Clear", "Copy"}; // 以下用以上的常數設定所需屬性 name = n; att = a; e = null; // null 表示指向虛無的參考 userinput = ""; result = ""; frame = new JFrame(); GUIComponent = new ArrayList<JComponent>(12); } // run() 為建立視窗的函數 public void run() { frame.setSize(600, 160); frame.setLayout(new GridBagLayout()); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); // 以下建立視窗元件 int i; for (i = 0; i < 3; i++) { JLabel nLabel = new JLabel(name[i]); GUIComponent.add(nLabel); } for (i = 0; i < 2; i++) { JTextField nText = new JTextField("", 32); GUIComponent.add(nText); } for (i = 3; i < 10; i++) { JButton nButton = new JButton(name[i]); GUIComponent.add(nButton); } for (i = 0; i < GUIComponent.size(); i++) { addComponent(i); } // 以下個視窗元件註冊所要處理的事件 JTextField t = (JTextField) GUIComponent.get(3); t.addActionListener(new InputListener()); t.addCaretListener(new InputListener()); t = (JTextField) GUIComponent.get(4); t.addActionListener(new OutputListener()); JButton b = (JButton) GUIComponent.get(5); b.addActionListener(new NewListener()); b = (JButton) GUIComponent.get(6); b.addActionListener(new LoadListener()); b = (JButton) GUIComponent.get(7); b.addActionListener(new SaveListener()); b = (JButton) GUIComponent.get(8); b.addActionListener(new EncodeListener()); b = (JButton) GUIComponent.get(9); b.addActionListener(new DecodeListener()); b = (JButton) GUIComponent.get(10); b.addActionListener(new ClearListener()); b = (JButton) GUIComponent.get(11); b.addActionListener(new CopyListener()); frame.setVisible(true); } // 設定 GridBagConstraints 的函數 private void addComponent(int i) { GridBagConstraints c = new GridBagConstraints(); int a[] = att[i]; c.gridx = a[0]; c.gridy = a[1]; c.gridwidth = a[2]; c.gridheight = a[3]; c.weightx = a[4]; c.weighty = a[5]; c.fill = a[6]; c.anchor = a[7]; frame.add(GUIComponent.get(i), c); } // 使用者輸入文字方塊的事件 class InputListener implements ActionListener, CaretListener { public void actionPerformed(ActionEvent event) { JTextField t2 = (JTextField) GUIComponent.get(3); userinput = t2.getText(); JLabel t1 = (JLabel) GUIComponent.get(2); t1.setText("This is Input textfield. Your input is \'" + userinput + "\'"); } public void caretUpdate(CaretEvent event) { JTextField t2 = (JTextField) GUIComponent.get(3); userinput = t2.getText(); JLabel t1 = (JLabel) GUIComponent.get(2); t1.setText("This is Input textfield. Your input is \'" + userinput + "\'"); } } // 輸出文字方塊的事件 class OutputListener implements ActionListener { public void actionPerformed(ActionEvent event) { JLabel t = (JLabel) GUIComponent.get(2); t.setText("This is Output textfield. Why do you do this?"); } } // 建立新 Encrypt 物件的事件 class NewListener implements ActionListener { public void actionPerformed(ActionEvent event) { e = new Encrypt(); JLabel t = (JLabel) GUIComponent.get(2); t.setText("This is New button. A new Encrypt object is built."); } } // 載入已儲存 Encrypt 物件檔案的事件 class LoadListener implements ActionListener { public void actionPerformed(ActionEvent event) { JLabel t1 = (JLabel) GUIComponent.get(2); JTextField t2 = (JTextField) GUIComponent.get(4); try { FileInputStream fs = new FileInputStream("encryptor.ser"); ObjectInputStream is = new ObjectInputStream(fs); e = (Encrypt) is.readObject(); is.close(); fs.close(); t1.setText("This is Load button. Encrypt object is loaded."); } catch (Exception ex) { ex.printStackTrace(); t1.setText("This is Load button. Encrypt object is not loaded."); } finally { t2.setText("after Load ....."); } } } // 儲存 Encrypt 物件檔案的事件 class SaveListener implements ActionListener { public void actionPerformed(ActionEvent event) { JLabel t1 = (JLabel) GUIComponent.get(2); JTextField t2 = (JTextField) GUIComponent.get(4); try { FileOutputStream fs = new FileOutputStream("encryptor.ser"); ObjectOutputStream os = new ObjectOutputStream(fs); os.writeObject(e); os.close(); fs.close(); t1.setText("This is Save button. Encrypt object is saved."); } catch (Exception ex) { ex.printStackTrace(); t1.setText("This is Save button. Encrypt object is not saved."); } finally { t2.setText("after Save ....."); } } } // 進行編碼的事件 class EncodeListener implements ActionListener { public void actionPerformed(ActionEvent event) { JLabel t1 = (JLabel) GUIComponent.get(2); JTextField t2 = (JTextField) GUIComponent.get(4); if (userinput == "") { t1.setText("This is Encode button. No input string!!"); } else { if (e == null) { t1.setText("This is Encode button. No Encrypt object!!"); } else { result = e.toEncode(userinput); t2.setText(result); t1.setText("This is Encode button. The result is above."); } } } } // 進行解碼的事件 class DecodeListener implements ActionListener { public void actionPerformed(ActionEvent event) { JLabel t1 = (JLabel) GUIComponent.get(2); JTextField t2 = (JTextField) GUIComponent.get(4); if (userinput == "") { t1.setText("This is Decode button. No input string!!"); } else { if (e == null) { t1.setText("This is Decode button. No Encrypt object!!"); } else { result = e.toDecode(userinput); t2.setText(result); t1.setText("This is Decode button. The result is above."); } } } } // 清楚文字方塊及暫存資料的事件 class ClearListener implements ActionListener { public void actionPerformed(ActionEvent event) { userinput = ""; result = ""; JTextField t2 = (JTextField) GUIComponent.get(3); t2.setText(""); JTextField t3 = (JTextField) GUIComponent.get(4); t3.setText(""); JLabel t1 = (JLabel) GUIComponent.get(2); t1.setText("This is Clear button. Your input is clear."); } } // 將輸出結果拷貝到作業系統的剪貼簿 class CopyListener implements ActionListener { public void actionPerformed(ActionEvent event) { JTextField t2 = (JTextField) GUIComponent.get(4); t2.selectAll(); t2.copy(); JLabel t1 = (JLabel) GUIComponent.get(2); t1.setText("This is Copy button. Result is copied to clipboard."); } } } /* 《程式語言教學誌》的範例程式 http://pydoing.blogspot.com/ 檔名:EncryptorGUI.java 功能:示範 Java 程式 作者:張凱慶 時間:西元 2011 年 4 月 */
您可以繼續參考
範例程式碼
相關目錄
回 Java 入門指南
回 Java 教材目錄
回首頁
參考資料
The JavaTM Tutorials: Getting Started
The JavaTM Tutorials: Learning the Java Language
The JavaTM Tutorials: Essential Classes
The Java Language Specification, Third Edition
本文於 2013 年 1 月訂正
沒有留言:
張貼留言