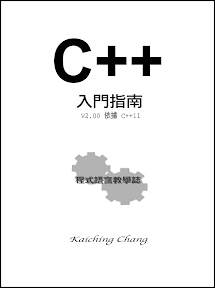
標準程式庫 (standard library) 是依據 C++ 標準,隨編譯器 (compiler) 提供的程式庫 (library)

我們已經用過不少標準程式庫中的例子,例如於命令列印出字串 (string)
018 | cout << m << endl; |
完整程式請參考單元 1 - 認識 C++ 語言。
或是產生擬隨機整數
020 | int a = rand() % 10; |
完整程式請參考單元 16 - 繼續測試。
標準程式庫涵蓋各方面的應用,包括在最新標準 C++11 內,計有以下的標頭檔 (header file)
容器 (container) 相關
- <array>
- <bitset>
- <deque>
- <forward_list>
- <list>
- <map>
- <queue>
- <set>
- <stack>
- <unordered_map>
- <unordered_set>
- <vector>
通用相關
- <algorithm>
- <chrono>
- <functional>
- <iterator>
- <memory>
- <stdexcept>
- <tuple>
- <utility>
地方化相關
- <locale>
- <codecvt>
字串相關
- <string>
- <regex>
串流及輸出入相關
- <fstream>
- <iomanip>
- <ios>
- <iosfwd>
- <iostream>
- <istream>
- <ostream>
- <sstream>
- <streambuf>
語言相關
- <exception>
- <limits>
- <new>
- <typeinfo>
執行緒 (thread) 相關
- <thread>
- <mutex>
- <condition_variable>
- <future>
數學相關
- <complex>
- <random>
- <valarray>
- <numeric>
以及 C 標準函數庫。
如果要好好、詳細的介紹,那可能就是一本大部頭的書了說。倒是須注意,若引入標準程式庫中的標頭檔,需要用 using 設定命名空間 (name space) ,不然就得加上命名空間與作用域運算子 (scope operator) :: ,例如下面的程式計算迴圈執行時間
001 | #include <ctime> |
002 | #include <iostream> |
003 | |
004 | int main() { |
005 | time_t t1 = time(NULL); |
006 | unsigned long i = 1000000000; |
007 | std::cout << i << std::endl; |
008 | |
009 | while (true) { |
010 | if (i == 0) { |
011 | break; |
012 | } |
013 | |
014 | i--; |
015 | } |
016 | |
017 | time_t t2 = time(NULL); |
018 | |
019 | std::cout << t2 - t1 << std::endl; |
020 | |
021 | return 0; |
022 | } |
023 | |
024 | /* time_test.cpp |
025 | Kaiching Chang |
026 | 2014-5 */ |
由於 cout 與 endl 都是屬於 std 定義的名稱,如果程式中沒有用
using namespace std; |
或
using std::cout; | |
using std::cin; | |
using std::endl; |
就得連帶 std:: 把完整名稱寫出來。
上例也引進標準程式庫中的 ctime ,用到裡頭的 time_t 型態與 time() 函數,因為 c 開頭的標準程式庫的標頭檔是從 C 語言延伸來的,由於 C 語言並沒有命名空間的概念,因此只要引進標頭檔就可直接使用裡頭定義的相關程式。
雖然 C++ 的標準程式庫提供非常豐富的資源,可是使用者介面 (user interface) 仍是沿襲 C 語言命令列 (command line) 的風格,因此我們要替 Encrypt 設計一個圖形使用者介面 (graphical user interface, GUI) 就得借助第三方程式庫 (third party library) 。所以接下來就讓我們繼續認識一下第三方程式庫 - Qt 吧!
中英文術語對照
標準程式庫 | standard library |
編譯器 | compiler |
程式庫 | library |
字串 | string |
標頭檔 | header file |
容器 | container |
執行緒 | thread |
命名空間 | name space |
作用域運算子 | scope operator |
使用者介面 | user interface |
命令列 | command line |
圖形使用者介面 | graphical user interface, GUI |
第三方程式庫 | third party library |
重點整理
- 標準程式庫是依 C++ 標準隨編譯器提供的程式庫,第三方程式庫則是其他開發商提供程式庫。
- 標準程式庫提供眾多的應用,包括容器、工具、地方資訊、字串、串流及輸出入、語言、執行緒、數學等應用。
- 如果沒有用 using 使用標準程式庫的名稱,就要連帶用標準程式庫的命名空間及作用域運算子 std:: 。
問題與討論
- 為什麼要有標準程式庫?不能程式的所有功能都自己開發嗎?
- 什麼是命名空間?為什麼要用命名空間?
- 為什麼要有第三方程式庫?除了 Qt 外還有其他的第三方程式庫嗎?
練習
- 寫一個程式 exercise2201.cpp ,利用標準程式庫中 string 的 string 與 algorithm 的 random_shuffle() ,攪亂字串 "0123456789" 的順序。
- 承上題,這樣攪亂後的順序都是固定的,如果要重新執行程式而有所更改的話,需要提供第三個隨機函數的參數給 random_shuffle() ,另寫一個程式 exercise2202.cpp ,利用標準程式庫中 ctime 的 time() 與 cstdlib 的 rand() 、 srand() 設計隨機函數 myrandom() 。
the end
沒有留言:
張貼留言