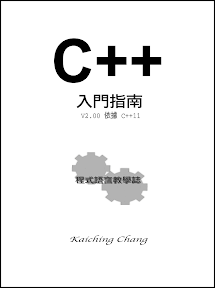
class_demo4.h
// Demo 類別的標頭檔 // 僅有 Demo 類別的宣告 class Demo { // 宣告 public 的成員 public: Demo(); Demo(int n1); Demo(int n1, int n2); void set_a(int n); void set_b(int n); int get_a(); int get_b(); int do_something(); // 宣告 private 的成員 private: int a; int b; }; /* 檔名: class_demo4.h 作者: Kaiching Chang 時間: 2014-5 */
class_demo4.cpp
// 須引進標頭檔 #include "class_demo4.h" // 沒有參數的建構函數 Demo::Demo() { set_a(1); set_b(1); } // 一個參數的建構函數 Demo::Demo(int n1) { set_a(n1); set_b(n1); } // 兩個參數的建構函數 Demo::Demo(int n1, int n2) { set_a(n1); set_b(n2); } int Demo::do_something() { // 改成呼叫 getter 成員函數 return get_a() + get_b(); } // setter 與 getter 成員函數 void Demo::set_a(int n) { a = n; } void Demo::set_b(int n) { b = n; } int Demo::get_a() { return a; } int Demo::get_b() { return b; } /* 檔名: class_demo4.cpp 作者: Kaiching Chang 時間: 2014-5 */
class_demo5.cpp
#include <iostream> // 須引進標頭檔 #include "class_demo4.h" using namespace std; int main(void) { Demo t1; Demo t2(11); Demo t3(11, 22); cout << endl; cout << t1.do_something() << endl; cout << t2.do_something() << endl; cout << t3.do_something() << endl; cout << endl; return 0; } /* 檔名: class_demo5.cpp 作者: Kaiching Chang 時間: 2014-5 */
exercise1301.h
class IntegerDemo { public: IntegerDemo(int); void set_value(int); int get_value(); void Add(int); void Subtract(int); void Multiply(int); void Divide(int); private: int value; }; /* 檔名: exercise1301.h 作者: Kaiching Chang 時間: 2014-5 */
exercise1301.cpp
#include <iostream> #include "exercise1301.h" using namespace std; IntegerDemo::IntegerDemo(int p) { set_value(p); } void IntegerDemo::set_value(int p) { value = p; } int IntegerDemo::get_value() { return value; } void IntegerDemo::Add(int p) { value += p; } void IntegerDemo::Subtract(int p) { value -= p; } void IntegerDemo::Multiply(int p) { value *= p; } void IntegerDemo::Divide(int p) { value /= p; } int main(void) { int input1; int input2; int input3; int input4; int input5; cin >> input1; cin >> input2; cin >> input3; cin >> input4; cin >> input5; cout << endl; IntegerDemo a(input1); cout << "a = " << a.get_value() << endl; a.Add(input2); cout << "a = " << a.get_value() << endl; a.Subtract(input3); cout << "a = " << a.get_value() << endl; a.Multiply(input4); cout << "a = " << a.get_value() << endl; a.Divide(input5); cout << "a = " << a.get_value() << endl; cout << endl; return 0; } /* 檔名: exercise1301.cpp 作者: Kaiching Chang 時間: 2014-5 */
exercise1302.h
class FactorialDemo { public: FactorialDemo(int); void set_value(int); int get_value(); private: int value; }; /* 檔名: exercise1302.cpp 作者: Kaiching Chang 時間: 2014-5 */
exercise1302.cpp
#include <iostream> #include "exercise1302.h" using namespace std; FactorialDemo::FactorialDemo(int p) { int result = 1; for (int i = 1; i <= p; i++) { result *= i; } set_value(result); } void FactorialDemo::set_value(int p) { value = p; } int FactorialDemo::get_value() { return value; } int main(void) { cout << endl; FactorialDemo a(10); cout << "10! = " << a.get_value() << endl; cout << endl; return 0; } /* 檔名: exercise1302.cpp 作者: Kaiching Chang 時間: 2014-5 */
exercise1303.h
class FibonacciDemo { public: FibonacciDemo(int); void set_value(int); int get_value(); private: int value; }; /* 檔名: exercise1303.h 作者: Kaiching Chang 時間: 2014-5 */
exercise1303.cpp
#include <iostream> #include "exercise1303.h" using namespace std; FibonacciDemo::FibonacciDemo(int n) { int f0 = 0; int f1 = 1; int f2; if (n == 0) { set_value(f0); } else if (n == 1) { set_value(f1); } else { for (int i = 2; i <= n; i++) { f2 = f0 + f1; f0 = f1; f1 = f2; } set_value(f2); } } void FibonacciDemo::set_value(int n) { value = n; } int FibonacciDemo::get_value() { return value; } int main(void) { cout << endl; FibonacciDemo a(10); cout << "f(10) = " << a.get_value() << endl; cout << endl; return 0; } /* 檔名: exercise1303.cpp 作者: Kaiching Chang 時間: 2014-5 */
the end
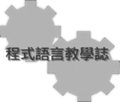
沒有留言:
張貼留言