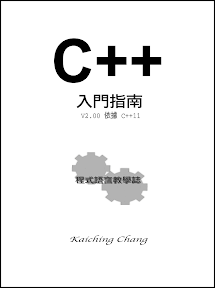
class_demo2.cpp
#include <iostream> using namespace std; class Demo { // 宣告 public 的成員 public: void set_a(int n); void set_b(int n); int get_a(); int get_b(); int DoSomething(); // 宣告 private 的成員 private: int a; int b; }; int Demo::DoSomething() { // 改成呼叫 getter 成員函數 return get_a() + get_b(); } // setter 與 getter 成員函數 void Demo::set_a(int n) { a = n; } void Demo::set_b(int n) { b = n; } int Demo::get_a() { return a; } int Demo::get_b() { return b; } int main(void) { Demo t; // 由呼叫 setter 設定成員變數 t.set_a(11); t.set_b(22); cout << endl; cout << t.DoSomething() << endl; cout << endl; return 0; } /* 檔名: class_demo2.cpp 作者: Kaiching Chang 時間: 2014-5 */
exercise1101.cpp
#include <iostream> using namespace std; class IntegerDemo { public: void set_value(int); int get_value(); void Add(int); void Subtract(int); void Multiply(int); void Divide(int); private: int value; }; void IntegerDemo::set_value(int p) { value = p; } int IntegerDemo::get_value() { return value; } void IntegerDemo::Add(int p) { value += p; } void IntegerDemo::Subtract(int p) { value -= p; } void IntegerDemo::Multiply(int p) { value *= p; } void IntegerDemo::Divide(int p) { value /= p; } int main(void) { int input1; int input2; int input3; int input4; int input5; cin >> input1; cin >> input2; cin >> input3; cin >> input4; cin >> input5; cout << endl; IntegerDemo a; a.set_value(input1); cout << "a = " << a.get_value() << endl; a.Add(input2); cout << "a = " << a.get_value() << endl; a.Subtract(input3); cout << "a = " << a.get_value() << endl; a.Multiply(input4); cout << "a = " << a.get_value() << endl; a.Divide(input5); cout << "a = " << a.get_value() << endl; cout << endl; return 0; } /* 檔名: exercise1101.cpp 作者: Kaiching Chang 時間: 2014-5 */
exercise1102.cpp
#includeusing namespace std; class FactorialDemo { public: void set_value(int); int get_value(); void Run(int); private: int value; }; void FactorialDemo::set_value(int p) { value = p; } int FactorialDemo::get_value() { return value; } void FactorialDemo::Run(int p) { int result = 1; for (int i = 1; i <= p; i++) { result *= i; } set_value(result); } int main(void) { cout << endl; FactorialDemo a; a.Run(10); cout << "10! = " << a.get_value() << endl; cout << endl; return 0; } /* 檔名: exercise1102.cpp 作者: Kaiching Chang 時間: 2014-5 */
exercise1103.cpp
#include <iostream> using namespace std; class FibonacciDemo { public: void set_value(int); int get_value(); void Run(int); private: int value; }; void FibonacciDemo::set_value(int n) { value = n; } int FibonacciDemo::get_value() { return value; } void FibonacciDemo::Run(int n) { int f0 = 0; int f1 = 1; int f2; if (n == 0) { set_value(f0); } else if (n == 1) { set_value(f1); } else { for (int i = 2; i <= n; i++) { f2 = f0 + f1; f0 = f1; f1 = f2; } set_value(f2); } } int main(void) { cout << endl; FibonacciDemo a; a.Run(10); cout << "f(10) = " << a.get_value() << endl; cout << endl; return 0; } /* 檔名: exercise1103.cpp 作者: Kaiching Chang 時間: 2014-5 */the end
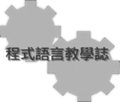
沒有留言:
張貼留言