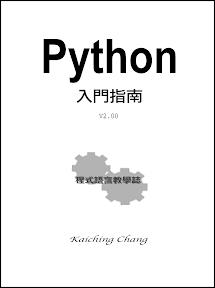
library_demo.py
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 | import time import datetime now = datetime.datetime.now() year = datetime.date.today(). strftime ( "%Y" ) month = datetime.date.today(). strftime ( "%B" ) wday = datetime.date.today(). strftime ( "%A" ) print( "Current date and time: " , now) print( "Current year: " , year) print( "Month of the year: " , month) print( "Day of the week: " , wday) # 檔名: library_demo.py # 作者: Kaiching Chang # 時間: July, 2014 |
library_demo2.py
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | import re str = "There is no spoon." v1 = re.sub(r "a" , "e" , str) v2 = re.sub(r "e" , "i" , v1) v3 = re.sub(r "i" , "o" , v2) v4 = re.sub(r "o" , "u" , v3) v5 = re.sub(r "u" , "a" , v4) print(v5) # 檔名: library_demo2.py # 作者: Kaiching Chang # 時間: July, 2014 |
library_demo3.py
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 | from decimal import * print() print(round(0.7 * 1.05, 2)) print(round(Decimal( "0.7" ) * Decimal( "1.05" ), 2)) print() print(1.00 % 0.10) print(Decimal( "1.00" ) % Decimal( "0.10" )) print() print(1 / 7) print(Decimal( "1" ) / Decimal( "7" )) print() # 檔名: library_demo3.py # 作者: Kaiching Chang # 時間: July, 2014 |
guessgame.py
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 | from random import shuffle class GuessGame: def __init__(self, digit=None): if digit == None or digit < 3 or digit > 6: self.length = 4; else : self.length = digit; self.set_game(); def set_game(self): while True: self.answer = [chr(i) for i in range(48, 58)] shuffle(self.answer) self.answer = self.answer[0:self.length] if self.answer[0] != "0" : break self.times = 0 self.a = 0 self.b = 0 def find_number(self, array): for i in array: if array.count(i) > 1: return True return False def ab_counter(self, guess): self.a = 0 self.b = 0 for i in guess: if i in self.answer: if guess.index(i) == self.answer.index(i): self.a += 1 else : self.b += 1 def run(self): self.times = 0 while True: self.times += 1 guess = list(input( ": " )) if len(guess) != self.length: print( "Wrong length!!" ) continue if self.find_number(guess): print( "Repeating digits!!" ) continue self.ab_counter(guess) if self.a == self.length: print( "Congratulation!!" ) break else : print(str(self.a) + "A" + str(self.b) + "B" ) print( "You guess " + str(self.times) + " times." ) if __name__ == "__main__" : g = GuessGame() g.run() # 檔名: guessgame.py # 作者: Kaiching Chang # 時間: July, 2014 |
the end
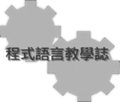
沒有留言:
張貼留言