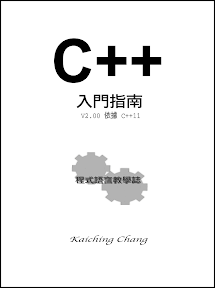
main.cpp
1 2 3 4 5 6 7 8 9 10 11 | #include "gamewindow.h" #include <QApplication> int main( int argc, char *argv[]) { QApplication a(argc, argv); GameWindow w; w.show(); return a.exec(); } |
encryptwindow.h
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 | #ifndef ENCRYPTWINDOW_H #define ENCRYPTWINDOW_H #include <QMainWindow> namespace Ui { class EncryptWindow; } class EncryptWindow : public QMainWindow { Q_OBJECT public : explicit EncryptWindow(QWidget *parent = 0); ~EncryptWindow(); private : Ui::EncryptWindow *ui; }; #endif // ENCRYPTWINDOW_H |
exercise2501.h
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 | #include <string> using namespace std; // 宣告 GuessGame 類別 class GuessGame { public : // 宣告建構函數 GuessGame( int ); // 存取函數與修改函數 void set_game(); string get_answer(); int get_times(); int get_a(); int get_b(); // 工具函數 bool FindNumber(string, int , char ); void ABCounter(string, string); // 命令列遊戲版本 void Run(); private : // 資料成員區 string answer; int length; string guess; int a; int b; int times; }; /* 檔名: exercise2501.h 作者: Kaiching Chang 時間: 2014-5 */ |
exercise2501.cpp
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 | #include <cstdlib> #include <ctime> #include <algorithm> #include <iostream> #include "exercise2501.h" int myrandom( int i) { return rand () % i; } GuessGame::GuessGame( int digit) { if (digit < 3 || digit > 6) { length = 4; } else { length = digit; } set_game(); } void GuessGame::set_game() { srand ( time (0)); string result = "0123456789" ; while ( true ) { random_shuffle(result.begin(), result.end(), myrandom); if (result.at(0) != '0' ) { break ; } } answer = result.substr(0, length); times = 0; a = 0; b = 0; } string GuessGame::get_answer() { return answer; } int GuessGame::get_times() { return times; } int GuessGame::get_a() { return a; } int GuessGame::get_b() { return b; } int main() { GuessGame g(4); cout << g.get_answer() << endl; return 0; } /* 檔名: exercise2501.cpp 作者: Kaiching Chang 時間: 2014-5 */ |
the end
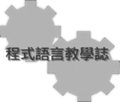
沒有留言:
張貼留言