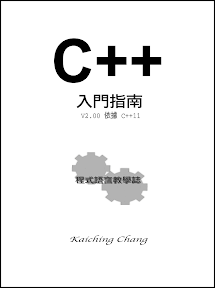
encrypt.cpp
// 引入標準程式庫中的 cstdlib 及 ctime #include <cstdlib> // srand(), rand() #include <ctime> // time() // 引入 Encrypt 類別的標頭檔 #include "encrypt.h" // Encrypt 的建構函數 Encrypt::Encrypt() { // 呼叫 setter 設定 code_array set_code_array(); } // 設定 code_array 的 setter 成員函數 void Encrypt::set_code_array() { // 設定擬隨機數的種子 srand(time(0)); // 取得 a 、 b 值 int a = 0; int b = 0; while (a % 2 == 0) { a = rand() % 10; b = rand() % 10; } // 利用公式建立密碼表字串 int x, y, m; char r; char c = 'a'; string s; int i; for (i = 0; i < N; i++) { x = c; y = x * a + b; m = y % N; s += (char) m + DIFF; c++; } // 將建立好的密碼表直接設定給成員變數 code_array = s; } // 由參數字串設定密碼表 void Encrypt::set_code_array(string s) { code_array = s; } // 回傳密碼表字串的 getter 成員函數 string Encrypt::get_code_array() { return code_array; } // 進行編碼工作的成員函數 string Encrypt::ToEncode(string s) { // 由參數字串取得字元的暫存變數 char c; // 暫存編碼結果的字串 string r; int i, m; // 利用迴圈走完參數字串的所有字元 for (i = 0; i < s.size(); i++) { // 判斷該字元是否為英文小寫字母 // 若是英文小寫字母就進行編碼轉換 if (s.at(i) >= DIFF && s.at(i) < DIFF + N) { c = s.at(i); m = c - 97; r += get_code_array().at(m); } else { r += s.at(i); } } // 結束回傳編碼過的字串 return r; } // 進行解碼工作的成員函數 string Encrypt::ToDecode(string s) { // 暫存解碼結果的字串 string r; int i, j; // 第一層迴圈逐一取得每一個字元 for (i = 0; i < s.size(); i++) { // 判斷該字元是否為英文小寫字母 // 若是英文小寫字母就進行解碼轉換 if (s.at(i) >= DIFF && s.at(i) < DIFF + N) { // 第二層迴圈尋找該字元在密碼表中的索引值 // 該索引值加上 DIFF 就可轉換回原本的字元 for (j = 0; j < N; j++) { if (s.at(i) == get_code_array().at(j)) { r += (char) j + DIFF; break; } } } else { r += s.at(i); } } // 結束回傳解碼過的字串 return r; } /* encrypt.cpp Kaiching Chang 2014-5 */
encrypt_demo.cpp
// 引入標準程式庫中的 iostream #include <iostream> // 使用 std 中的兩個名稱 using std::cout; // 標準輸出串流的物件 using std::endl; // 新行符號,等於 '\n' // 引入 Encrypt 類別的標頭檔 #include "encrypt.h" // 程式執行的 main() 函數 int main() { // 印出空白一行 cout << endl; // 建立 Encrypt 物件 Encrypt encryptor; // 印出密碼表字串 cout << encryptor.get_code_array() << endl << endl ; // 印出準備編碼的字串 string d = "There is no spoon."; cout << d << endl; // 將字串編碼,然後印出編碼結果 string r1 = encryptor.ToEncode(d); cout << r1 << endl << endl; // 重新解碼碼,然後印出解碼結果 string r2 = encryptor.ToDecode(r1); cout << r2 << endl << endl; return 0; } /* encrypt_demo.cpp Kaiching Chang 2014-5 */
exercise1901.cpp
#include <iostream> #include <cmath> #include <cstdlib> #include <ctime> using namespace std; // 將整數拆成整數陣列的函數 void ArrayNumber(int array[], int number, int length) { int i; int j = pow(10, length - 1); int temp = number; for (i = 0; i < length; i++) { array[i] = temp / j; temp %= j; j /= 10; } } // 計算 A 、 B 值 void ABCounter(int array1[], int array2[], int array3[], int length) { int a = 0; int b = 0; for (int i = 0; i < length; i++) { for (int j = 0; j < length; j++) { if (array1[i] == array2[j]) { if (i == j) { a++; } else { b++; } } } } array3[0] = a; array3[1] = b; } // 找尋陣列中是否已有指定數字 bool FindNumber(int array[], int length, int index, int number) { for (int i = 0; i < length; i++) { if (i == index) { continue; } if (array[i] == number) { return true; } } return false; } // 建立猜數字遊戲答案的函數 int RandomNumber(int array[], int length) { srand(time(0)); int m; int i = 0; int base = pow(10, length - 1); int result = 0; // 建立不重複的四位數 while (i < length) { m = rand() % 10; // 若第一位數為 0 則重來 if (i == 0 && m == 0) { continue; } // 將答案儲存在陣列 array[i] = m; // 有重複,這一輪重來 if (FindNumber(array, length, i, m)) { continue; } // 沒重複,將該數字加進四位數之中 else { result += m * base; base /= 10; i++; } } return result; } int main(void) { // 建立答案 int answer_array[4]; int answer = RandomNumber(answer_array, 4); int times = 0; while (true) { // 計算次數 times++; // 接受猜測 int guess; cin >> guess; int guess_array[4]; ArrayNumber(guess_array, guess, 4); // 計算 A 、 B 值 int ab_array[2]; ABCounter(answer_array, guess_array, ab_array, 4); // 判斷使用者是否猜對 if (answer == guess) { cout << "Right!! You guess " << times << " times!!" << endl; break; } else { cout << "Wrong!! " << ab_array[0] << "A" << ab_array[1] << "B!!" << endl; } } } } /* 檔名: exercise1901.cpp 作者: Kaiching Chang 時間: 2014-5 */
exercise1902.cpp
#include <iostream> #include <cmath> #include <cstdlib> #include <ctime> using namespace std; // 將整數拆成整數陣列的函數 void ArrayNumber(int array[], int number, int length) { int i; int j = pow(10, length - 1); int temp = number; for (i = 0; i < length; i++) { array[i] = temp / j; temp %= j; j /= 10; } } // 計算 A 、 B 值 void ABCounter(int array1[], int array2[], int array3[], int length) { int a = 0; int b = 0; for (int i = 0; i < length; i++) { for (int j = 0; j < length; j++) { if (array1[i] == array2[j]) { if (i == j) { a++; } else { b++; } } } } array3[0] = a; array3[1] = b; } // 找尋陣列中是否已有指定數字 bool FindNumber(int array[], int length, int index, int number) { for (int i = 0; i < length; i++) { if (i == index) { continue; } if (array[i] == number) { return true; } } return false; } // 建立猜數字遊戲答案的函數 int RandomNumber(int array[], int length) { srand(time(0)); int m; int i = 0; int base = pow(10, length - 1); int result = 0; // 建立不重複的四位數 while (i < length) { m = rand() % 10; // 若第一位數為 0 則重來 if (i == 0 && m == 0) { continue; } // 將答案儲存在陣列 array[i] = m; // 有重複,這一輪重來 if (FindNumber(array, length, i, m)) { continue; } // 沒重複,將該數字加進四位數之中 else { result += m * base; base /= 10; i++; } } return result; } int main(void) { // 建立答案 int answer_array[4]; int answer = RandomNumber(answer_array, 4); int times = 0; while (true) { // 計算次數 times++; // 接受猜測 int guess; cin >> guess; int guess_array[4]; ArrayNumber(guess_array, guess, 4); // 檢查使用者是否輸入四個不同數字 bool state = false; for (int i = 0; i < 4; i++) { if (FindNumber(guess_array, 4, i, guess_array[i])) { state = true; } } if (state) { cout << "Please guess 4 different numbers!!" << endl; continue; } // 計算 A 、 B 值 int ab_array[2]; ABCounter(answer_array, guess_array, ab_array, 4); // 判斷使用者是否猜對 if (answer == guess) { cout << "Right!! You guess " << times << " times!!" << endl; break; } else { cout << "Wrong!! " << ab_array[0] << "A" << ab_array[1] << "B!!" << endl; } } } /* 檔名: exercise1902.cpp 作者: Kaiching Chang 時間: 2014-5 */
exercise1903.cpp
#include <iostream> #include <cmath> #include <cstdlib> #include <ctime> using namespace std; // 將整數拆成整數陣列的函數 void ArrayNumber(int array[], int number, int length) { int i; int j = pow(10, length - 1); int temp = number; for (i = 0; i < length; i++) { array[i] = temp / j; temp %= j; j /= 10; } } // 計算 A 、 B 值 void ABCounter(int array1[], int array2[], int array3[], int length) { int a = 0; int b = 0; for (int i = 0; i < length; i++) { for (int j = 0; j < length; j++) { if (array1[i] == array2[j]) { if (i == j) { a++; } else { b++; } } } } array3[0] = a; array3[1] = b; } // 找尋陣列中是否已有指定數字 bool FindNumber(int array[], int length, int index, int number) { for (int i = 0; i < length; i++) { if (i == index) { continue; } if (array[i] == number) { return true; } } return false; } // 建立猜數字遊戲答案的函數 int RandomNumber(int array[], int length) { srand(time(0)); int m; int i = 0; int base = pow(10, length - 1); int result = 0; // 建立不重複的四位數 while (i < length) { m = rand() % 10; // 若第一位數為 0 則重來 if (i == 0 && m == 0) { continue; } // 將答案儲存在陣列 array[i] = m; // 有重複,這一輪重來 if (FindNumber(array, length, i, m)) { continue; } // 沒重複,將該數字加進四位數之中 else { result += m * base; base /= 10; i++; } } return result; } int main(void) { // 建立答案 int answer_array[4]; int answer = RandomNumber(answer_array, 4); int times = 0; while (true) { // 計算次數 times++; // 接受猜測 int guess; cin >> guess; int guess_array[4]; ArrayNumber(guess_array, guess, 4); // 檢查使用者是否輸入四個不同數字 bool state = false; for (int i = 0; i < 4; i++) { if (FindNumber(guess_array, 4, i, guess_array[i])) { state = true; } } if (state) { cout << "Please guess 4 different numbers!!" << endl; continue; } // 檢查使用者是否輸入過少或過多的數字 if (guess <= 999 || guess >= 10000) { cout << "Please input 4 numbers!!" << endl; continue; } // 計算 A 、 B 值 int ab_array[2]; ABCounter(answer_array, guess_array, ab_array, 4); // 判斷使用者是否猜對 if (answer == guess) { cout << "Right!! You guess " << times << " times!!" << endl; break; } else { cout << "Wrong!! " << ab_array[0] << "A" << ab_array[1] << "B!!" << endl; } } } /* 檔名: exercise1902.cpp 作者: Kaiching Chang 時間: 2014-5 */
the end
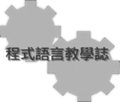
沒有留言:
張貼留言