C++ 速查手冊 V1.00 - 單元 5 範例
cpp-example05 C++教學, C++速查手冊

u05_1.cpp
#include <iostream> int main() { int a = 22; int b = 34; if (a > b) { std::cout << "Good luck!" << std::endl; } if (a != b) { std::cout << "Have a nice day!" << std::endl; } return 0; } /* Kaiching Chang u05_1.cpp 2014-02 */
u05_2.cpp
#include <iostream> int main() { int i; for (i = 0; i <= 10; i++) { std::cout << i << std::endl; } return 0; } /* Kaiching Chang u05_2.cpp 2014-02 */
u0501_1.cpp
#include <iostream> int main() { char a = 'h'; char b = 'h'; if (a == b) { std::cout << "Welcome to my world!" << std::endl; } return 0; } /* Kaiching Chang u0501_1.cpp 2014-02 */
u0501_2.cpp
#include <iostream> int main() { char a = 'h'; char b = 'k'; if (a == b) { std::cout << "Welcome to my world!" << std::endl; } if (a != b) { std::cout << "Free your mind." << std::endl; } return 0; } /* Kaiching Chang u0501_2.cpp 2014-02 */
u0502_1.cpp
#include <iostream> int main() { char a = 'h'; char b = 'k'; if (a == b) { std::cout << "Welcome to my world!" << std::endl; } else { std::cout << "How do you do?" << std::endl; } return 0; } /* Kaiching Chang u0502_1.cpp 2014-02 */
u0502_2.cpp
#include <iostream> int main() { char a = 'h'; char b = 'k'; if (a == 'a') { std::cout << "Yes!" << std::endl; } else { if (a == b) { std::cout << "No!" << std::endl; } else { std::cout << "What?" << std::endl; } } return 0; } /* Kaiching Chang u0502_2.cpp 2014-02 */
u0503_1.cpp
#include <iostream> int main() { int data[] = {1, 0 ,0, 1, 0, 1, 1, 0, 1, 1, 1, 0, 0, 0, 1, 1}; int one_count = 0; int zero_count = 0; int i; for (i = 0; i <= 15; i++) { switch (data[i]) { case 0: zero_count++; break; case 1: one_count++; break; } } std::cout << "There are " << zero_count; std::cout << " 0s, and " << one_count; std::cout << " 1s in data." << std::endl; return 0; } /* Kaiching Chang u0503_1.cpp 2014-02 */
u0503_2.cpp
#include <iostream> int main() { char saying[] = {'N', 'e', 'v', 'e', 'r', ' ', 'p', 'u', 't', ' ', 'o', 'f', 'f', ' ', 't', 'i', 'l', 'l', ' ', 't', 'o', 'm', 'o', 'r', 'r', 'o', 'w', ' ', 'w', 'h', 'a', 't', ' ', 'y', 'o', 'u', ' ', 'c', 'a', 'n', ' ', 'd', 'o', ' ', 't', 'o', 'd', 'a', 'y', '.'}; int aV, eV, iV, oV, uV, other, i; aV = eV = iV = oV = uV = 0; other = i = 0; while (saying[i] != '\0') { switch(saying[i]) { case 'A': case 'a': aV++; break; case 'E': case 'e': eV++; break; case 'I': case 'i': iV++; break; case 'O': case 'o': oV++; break; case 'U': case 'u': uV++; break; default: other++; break; } i++; } std::cout << "a: " << aV; std::cout << std::endl; std::cout << "e: " << eV; std::cout << std::endl; std::cout << "i: " << iV; std::cout << std::endl; std::cout << "o: " << oV; std::cout << std::endl; std::cout << "u: " << uV; std::cout << std::endl; std::cout << "other: " << other; std::cout << std::endl; return 0; } /* Kaiching Chang u0503_2.cpp 2014-02 */
u0504_1.cpp
#include <iostream> int main() { int sum = 0; int i; for (i = 1; i <= 100; i++) { sum += i; } std::cout << "1 + ... + 100 = " << sum << std::endl; return 0; } /* Kaiching Chang u0504_1.cpp 2014-02 */
u0504_2.cpp
#include <iostream> int main() { int i, j; for (i = 1; i <= 9; i++) { for (j = 1; j <= 9; j++) { std::cout << i * j << " "; } std::cout << std::endl; } return 0; } /* Kaiching Chang u0504_2.cpp 2014-02 */
u0504_3.cpp
#include <iostream> int main() { int array[] = {1, 2, 3, 4, 5}; for (int i: array) { std::cout << i << std::endl; } return 0; } /* Kaiching Chang u0504_3.cpp 2014-02 */
u0505_1.cpp
#include <iostream> int main() { int sum = 0; int i = 1; while (i <= 100) { sum += i; i++; } std::cout << "1 + ... + 100 = " << sum << std::endl; return 0; } /* Kaiching Chang u0505_1.cpp 2014-02 */
u0505_2.cpp
#include <iostream> int main() { int i = 1; int j = 1; while (i <= 9) { while (j <= 9) { std::cout << i * j << " "; j++; } j = 1; i++; std::cout << std::endl; } return 0; } /* Kaiching Chang u0505_2.cpp 2014-02 */
u0506_1.cpp
#include <iostream> int main() { int sum = 0; int i = 1; do { sum += i; i++; } while (i <= 100); std::cout << "1 + ... + 100 = " << sum << std::endl; return 0; } /* Kaiching Chang u0506_1.cpp 2014-02 */
u0506_2.cpp
#include <iostream> int main() { int i = 1; int j = 1; do { do { std::cout << i * j << " "; j++; } while (j <= 9); j = 1; i++; std::cout << std::endl; } while (i <= 9); return 0; } /* Kaiching Chang u0506_2.cpp 2014-02 */
u0507_1.cpp
#include <iostream> int main() { int data = 0; switch (data) { case 0: std::cout << "0" << std::endl; case 1: case 2: std::cout << "12" << std::endl; break; case 5: std::cout << "5" << std::endl; } return 0; } /* Kaiching Chang u0507_1.cpp 2014-02 */
u0507_2.cpp
#include <iostream> int main() { int i; for (i = 0; i < 10; i++) { if (i == 5) { break; } std::cout << i << std::endl; } return 0; } /* Kaiching Chang u0507_2.cpp 2014-02 */
u0508_1.cpp
#include <iostream> int main() { int i; for (i = 0; i < 10; i++) { if (i == 5) { continue; } std::cout << i << std::endl; } return 0; } /* Kaiching Chang u0508_1.cpp 2014-02 */
u0508_2.cpp
#include <iostream> int main() { int i = 0; while (i < 10) { if (i == 5) { continue; } std::cout << i << std::endl; i++; } return 0; } /* Kaiching Chang u0508_2.cpp 2014-02 */
u0509_2.cpp
#include <iostream> int main() { goto label_one; label_one: { std::cout << "Label One" << std::endl; goto label_two; } label_two: { std::cout << "Label Two" << std::endl; goto label_three; } label_three: { std::cout << "Label Three" << std::endl; } return 0; } /* Kaiching Chang u0509_2.cpp 2014-02 */
u0509_2.cpp
#include <iostream> int main() { int i = 1; if (i < 10) { goto label_one; } label_one: { std::cout << "Label One" << std::endl; goto label_three; } label_two: { std::cout << "Label Two" << std::endl; } label_three: { std::cout << "Label Three" << std::endl; i++; if (i < 10) { goto label_two; } } return 0; } /* Kaiching Chang u0509_2.cpp 2014-02 */
the end
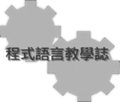
沒有留言:
張貼留言